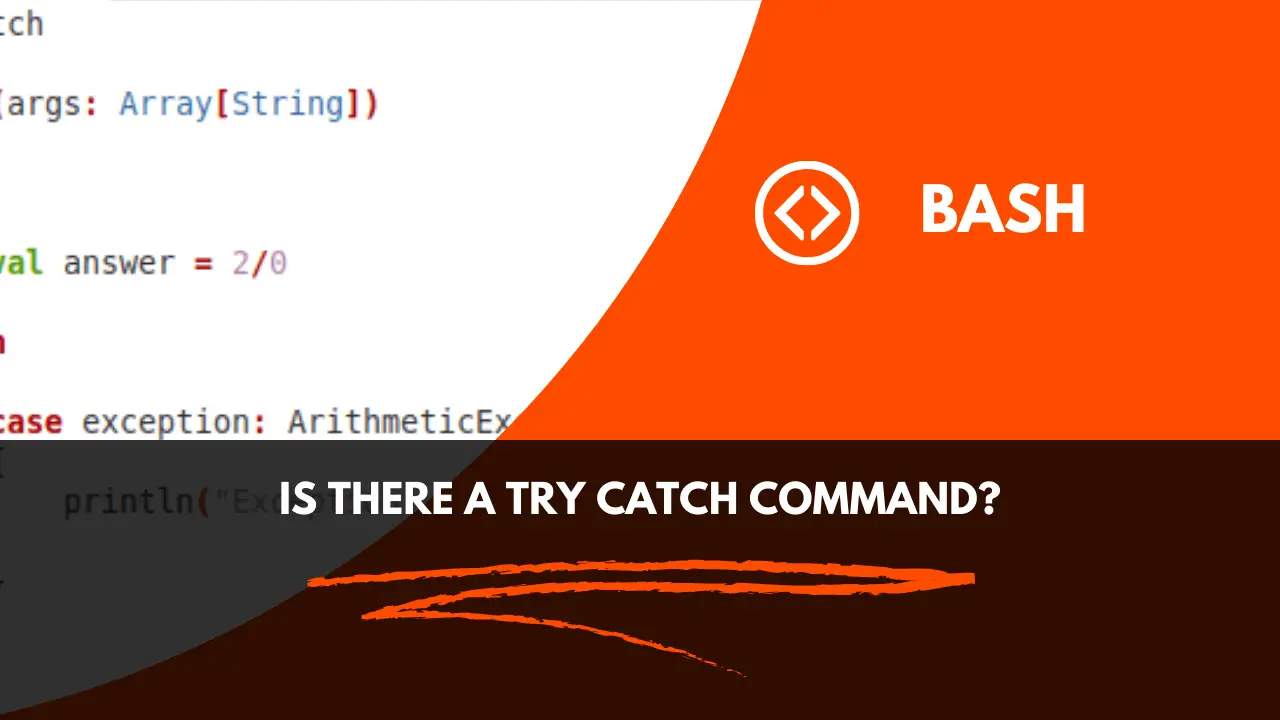
Introduction:
The “try/catch” command is not supported by “Bash”. Other applications for its properties exist, such “if/else” statements.
“Try-catch” is the programming word for handling exceptions. Stated simply, the “try” block throws an exception in the event of an error.
An exception like “file not found” can be routed to the “catch” section.
The “try/catch” command can be substituted with the methods discussed in this page.
How to Use TRY CATCH Command in Bash:
This section explains how to handle common mistakes in bash by using the try-catch command. We will go over six techniques in depth. Now, let’s get going.
Check the Exit Status
Examining the bash script’s exit status is the first approach. The exit status has the following syntax:
if ! some_command; then
echo "some_command returned an error"
fi
The built-in variable $? shows the state of the most recent bash command’s exit. For instance, you can confirm the bash script’s exit condition by combining $? with the if/else block.
Press “Ctrl + Alt + T” to launch the terminal and start this stage. Next, launch the preferred editor, such as Vim or Nano:
nano script.sh
vim script.sh
Add the following code in the bash script file:
# run some command
status=$?
if [ $status -eq 1 ]; then
echo "General error"
elif [ $status -eq 2 ]; then
echo "Misuse of shell builtins"
elif [ $status -eq 126 ]; then
echo "Command invoked cannot execute"
elif [ $status -eq 128 ]; then
echo "Invalid argument"
fi
Now, you can replace the #run some command
comment with any correct or wrong command to view the different error logs.
Use OR and AND Operator:
Another method you can opt for is the usage of AND and OR operators in bash scripts. To elaborate, use the ||
and &&
between two commands from the terminal or inside the bash script. Here is the syntax:
command1 || command2
command1 && command2
The ||
operator executes command 2 if command 1 fails. On the other hand, the &&
operator runs command 2 if and only if command 1 executes successfully.
For example, if you want to print something on the terminal only if the update has been completed successfully, then write:
sudo apt update && echo “Success”
The output looks like this:

Exit on Errors in Bash:
The try-catch command in Bash can also be used to quit a script upon encountering an error. The bash script by default keeps running even when it encounters an error. The script continues to run even after the error is written to stderr.
Nevertheless, error-handling techniques are not permitted by this default behavior. Use the set command in Bash to run the error mechanism’s exit. For instance, the syntax to add the exit on error code to a bash script is as follows:
set -e
#
# Some critical code blocks where no error is allowed
#
set +e
In this instance, if any of the earlier commands leave with a non-zero status, the set command causes bash to stop the execution immediately. Errors are the sole reason for the non-zero status. The status is always set to 0 otherwise.
For instance, to test the wrong command, type:
set -e
#
eho “This is a test code”
#
set +e
In addition, you can also use the set
command with the pipeline. The default pipeline behavior only returns a non-zero exit status if the last command in the pipeline fails. So any error that occurs between the different commands in the pipe is not reflected outside the pipe.
How to Make the “trap” Command Function as TRY CATCH?
When the user presses “CTRL+C” to stop the program, the “trap” command operates according to the signals that are provided to it by the operating system. It is a trigger that acts in accordance with a particular directive. The script below, for instance, continues to run until the user hits “CTRL+C.” When it is pressed, the screen reads “trap worked,” then it sleeps for “5” seconds before returning control to the user:
#!/bin/bash
trap ‘echo “trap worked”‘ INT
(
trap ” INT
sleep 5
echo “done”
) &
wait
The above script is named “script.sh.” Let’s execute it to view the results:
#!/bin/bash
function finish {
echo “service started”
sudo service mysql start
}
trap finish EXIT
echo “service stopped”
sudo service mysql stop
The script is named “script.sh”. Let’s execute it to view the output:
It first stops the service and then restarts it, as can be seen in the terminal above. Press “CTRL+C” to resume the service immediately after it has been interrupted.
The “try/catch” approach and the aforementioned examples are comparable in that a script with many instructions executes slowly. The message printed by the “echo” command will not be displayed, but you can remove it with the shortcut key combination “CTRL+Z.” However, using the “trap” command makes it simpler to determine which commands are effective and which are not.
How to Force Exit When Error is Detected in Bash?
To quit, use “set” in conjunction with “Brexit” or “-e” in bash. What it does is, in the event of an error, automatically terminate the command. This option tells “Bash” to stop the script right away if any command returns a non-zero exit status, which means there was a mistake.
#!/bin/bash
sudo apt-get update
sudo apt install git curl python3-pip
git clone https://github.com/example/repo.git
pip3 install -r requirements.txt
python3 app.py
It is named “script. sh”. To execute it, apply the below-stated command, as discussed:
The “Username” and “Password” for GitHub that were entered above are incorrect, which will result in an error and the script terminating as shown below:
As seen above, the script is immediately terminated once an error is popped.
Conclusion:
In contrast to the majority of other coding languages, bash scripting does not support the “try/catch” statement. However, there are other, possibly more valuable ways to do the same objective, such as checking the “exit status.” The script can also be ended instantly if an error occurs by using the “set -e” command.
The current state of the “try/catch” command in Bash and its substitutes were covered in this tutorial.
Come along with us to learn more about Linux.
stay on our website linuxhints.info
Get more information about
Reading and Writing CSV in Bash