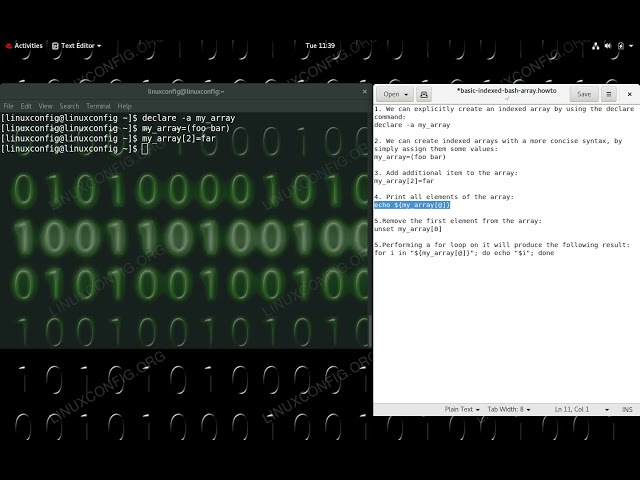
Introduction:
Are you looking for a tutorial on How to Find the Array Length in Bash? Then this guide is for you.
Multiple values can be stored in a single variable by using arrays.It’s incredibly easy to forget how many elements are in a given array because there is no upper limit to the number of elements you may keep in an array.
The good news is that determining an array’s length is not too difficult. An array’s length shows how many elements are contained within it. There are several ways to find the length, including printing the indices, loops, and the # symbol.
We’ll go over every approach there is for determining the array length in Bash. Now let’s get going!
How to Find the Array Length in Bash
We’ll go over each of the several ways to accomplish this so you can select the one that feels most natural to you. Let’s prepare our setup first.
Use this command to create a Bash script file:
$ touch arrlength.sh
Then make the file executable with this command:
$ chmod u+x arrlength.sh
Now launch your preferred text editor and open the Bash script. In this tutorial, we will utilize Nano. Use the following command to launch the script in Nano:
$ nano arrlength.sh
Now you’re ready to start writing some Bash commands. Follow along as we see how you can find array lengths in Bash.
Get Array Length Using the # Symbol
This is by far the simplest way to do this. Using the #
symbol, you can get the array length with only one line of code. Let us show you how. Look at the below example:
#! /bin/bash
distro=('ubuntu' 'linuxmint' 'debian' 'kali')
echo "The length of the array is: ${#distro[@]}"
Once you’ve written the script, save it with ‘Ctrl + O’ and exit from nano using ‘Ctrl + X’. Now run the script with this command:
$ ./arrlength.sh
As you can see from the above screenshot, the array length is 4, which is correct.
The unique syntax ${#array[@]} in Bash lets you retrieve an array’s length.The length of the array’s first element, in this example 6, is provided by ${#array}. Instead of only getting the first element, you may get the length of the entire array by adding [@] to the syntax.To specify every element in the array, use [@].
Get Array Length by Retrieving Array Indices
Obtaining the index numbers of each member in the array is a further method for determining the length of Bash arrays. With the use of the! symbol, we may obtain the index numbers.
But you should be aware that in Bash, an array’s index begins at 0. This implies that the length of the array can be found by adding 1 to the index of the final entry. You’ll understand it better when you watch this example:
#! /bin/bash
distro=('ubuntu' 'linuxmint' 'debian' 'kali')
echo "The indices of the elements of the array: ${!distro[@]}"
Save the script and exit. Then run the script with this command:
$ ./arrlength.sh
The index of the last element is 3. If you add 1 to it, it becomes 4, which is indeed the length of the array. Since indexing starts from 0, we increase the last index by one to get the length of the array.
Get the Array Length Using a Loop
Programmers with a lot of experience may be accustomed to iterating through arrays. This approach is suited you if you like to use your programming skills in that way.
To cycle through each element of the array, we employ a loop. We maintain track of the array’s total number of elements as we navigate through it. That is saved as a variable for later printing. Let’s examine an illustration:
#! /bin/bash
distro=('ubuntu' 'linuxmint' 'debian' 'kali')
# Initialize a counter
length=0
# Iterate over the array elements
for element in "${distro[@]}"; do
((length++))
done
# Print the length
echo "The length of the array is: $length"
Save the exit. Then run the script with this command:
$ ./arrlength.sh
Take a variable and set its initial value to 0. We raise the value of that variable by one every time we iterate through the array in the loop. The variable’s value will equal the array’s element count once we’ve finished traversing. The variable is then simply printed.
This method is useful if you forget the special syntax of the previous methods. You can use your loop knowledge to get the array size.
Get the Array Length Using the “wc” Command
The number of lines, words, characters, and bytes in a file can be counted with the wc Bash program. The array’s size can be found by counting the words in the string that results from converting the array to a string. Look at this illustration:
#! /bin/bash
distro=('ubuntu' 'linuxmint' 'debian' 'kali')
# Convert array to string
array_string="${distro[@]}"
# Count the number of words in the string
length=$(echo "$array_string" | wc -w)
# Print the length
echo "The length of the array is: $length"
Save the script, exit from the editor, and then run it with this command:
$ ./arrlength.sh
Everything functions as it should. Thus, we are translating our array into a string at this point. We use wc -w to count the words in the text. The -w flag is employed in word counting. The word count is then printed after being saved in a variable. The manual page for this command has additional information.
The requirement that no space character appear in any element of the array is a drawback of this technique. You will then receive the incorrect length. Let’s put it this way:
#! /bin/bash
distro=('ubuntu' 'linuxmint' 'debian' 'kali' 'pop os')
# Convert array to string
array_string="${distro[@]}"
# Count the number of words in the string
length=$(echo "$array_string" | wc -w)
# Print the length
echo "The length of the array is: $length"
Upon running the script, this is what we see:
The length should’ve been 5 since we added a single element. However, due to the space in ‘pop os’, they are counted as two separate words in the string. So we get an additional word when counting the length
Conclusion:
This guide shows you how to find the array length in Bash. We’ve covered many possible methods of doing it. Feel free to use any that you find best. If you have any questions about any of the methods, feel free to let us know in the comments down below.