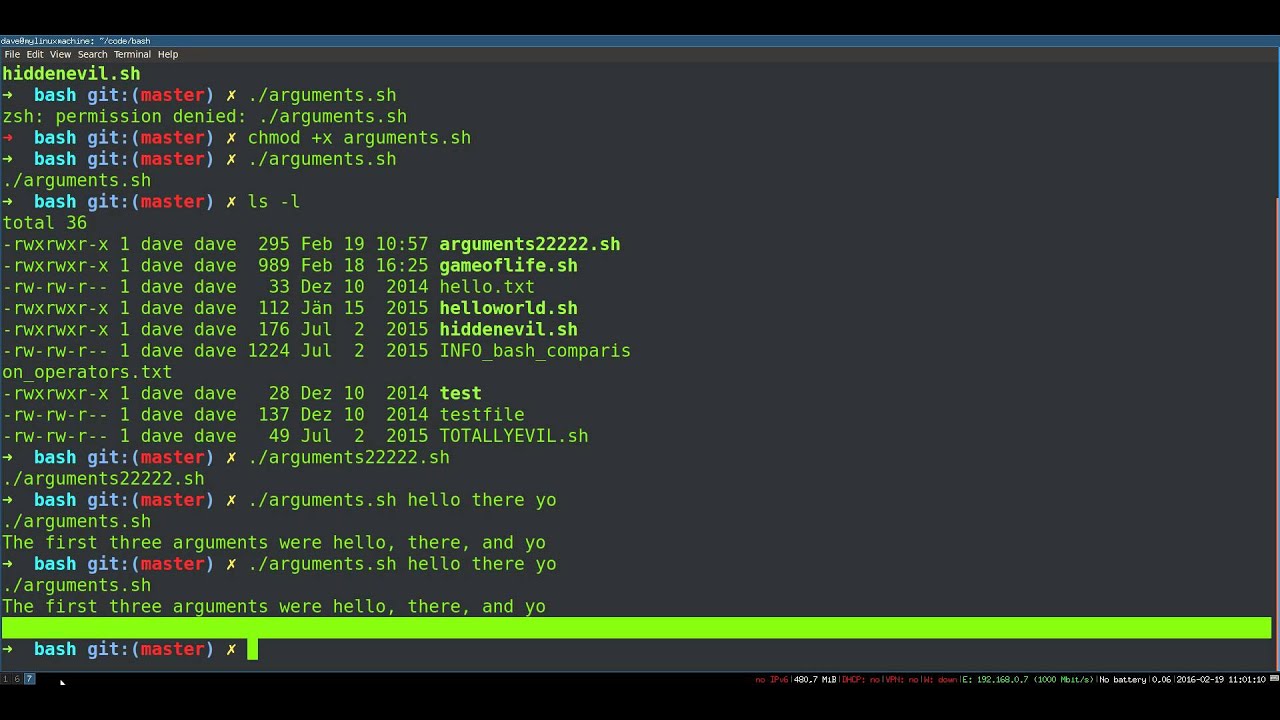
1. Overview
The many methods for determining whether Input Argument in a Bash Script will be covered in this quick lesson. We’ll use Bash for our examples, of course, but there may be a few minor variations with other shells.
2. Why Check the Existence of Input Arguments
When utilizing input arguments in a Bash script, we often assume that they will always be provided at the time the script is invoked. That isn’t always the case, though. Occasionally, the person calling the script might overlook one or more of the arguments, leading to erratic and unexpected behavior.
To make sure our code functions as intended, we should make sure input arguments are there and that the script contains their contents before utilizing any of them. Let’s look at the many approaches to that.
3. Check Whether No Arguments Are Supplied
Occasionally, we might overlook the arguments when calling a script.
Let’s construct a script that, in the event that there are no parameters, uses the exit command to leave the script with exit code 1 and verifies the number of input arguments:
#!/bin/bash
if [ "$#" -eq 0 ]
then
echo "No arguments supplied"
exit 1
fi
The number of input arguments the script gets is shown by the $# variable. The built-in test’s -eq parameter verifies that the values of its two operands are equal. If so, the requirement is satisfied.
4. Check if at Least One Argument Is Supplied
The script will quit if there isn’t at least one parameter, so let’s see how to check for that:
#!/bin/bash
if [ "$#" -lt 1 ]
then
echo "No arguments supplied"
exit 1
fi
The left operand’s value is compared to the right operand’s value using the -lt parameter in the test. If so, the requirement is satisfied. In this instance, we compare the number of arguments to 1 and leave the script with an exit status of 1 if the number of arguments is less than 1.
5. Check if the Input Arguments Count Is as Expected
We’ll look at how to determine whether the quantity of arguments provided matches the anticipated number.
Assume that there will be three arguments. Let’s now verify that the number of arguments supplied equals three and end the script if it doesn’t:
#!/bin/bash
if [ "$#" -ne 3 ]
then
echo "Incorrect number of arguments"
exit 1
fi
The test’s -ne parameter determines if the left operand’s value and the right operand’s value are equal. If so, the requirement is satisfied. In this instance, the script is terminated with an exit status of 1 after comparing the number of arguments to 3. If they are not equal.
6. Check if a Specific Argument Is Supplied
We’ll see how to check if a particular argument is present.
Let’s check whether the length of the first argument is non-zero:
#!/bin/bash
if [ -n "$1" ]
then
echo "First argument present"
fi
The -n test operator checks whether the given string operand has a non-zero length. If it does, then the expression returns true.
Now, let’s do a negative size check of the first argument and exit if it’s zero:
#!/bin/bash
if [ -z "$1" ]
then
echo "No argument supplied"
exit 1
fi
The -z test operator determines whether the operand, which is a string, has zero length. If it does, the function returns true, indicating that the length of our argument ($1) is zero.
7. Verifying the arguments
If a script is going to work with a file, you might want to check that the specified file exists before the script runs a command that is meant to process or examine it. For example:
filename=$1 # first argument should be filename if [ ! -e $filename ]; then echo “No such file: $filename” fi
The above commands! -e portion checks “if there is no file with the name.” A script can also be used to check the permissions of files. For instance, you can use commands like these to see if the user executing the script is able to read, write, or execute a file:
if [ ! -r $1 ]; then echo cannot read $1 fi if [ ! -x $1 ]; then echo cannot run $1 fi if [ ! -w $1 ]; then echo cannot write to $1 fi
The person running the script might see something like this:
$ countlines thatfile cannot read thatfile
8. Conclusion
We’ve covered how to check for input arguments inside a Bash script during runtime in this article.
We can determine whether to continue running the script by counting the number of arguments received by combining the test operator with $#. Additionally, we may determine whether to continue running the script by combining the positional command-line arguments ($1, $2, $3, etc.) with the test operator to see if the script receives a particular argument.